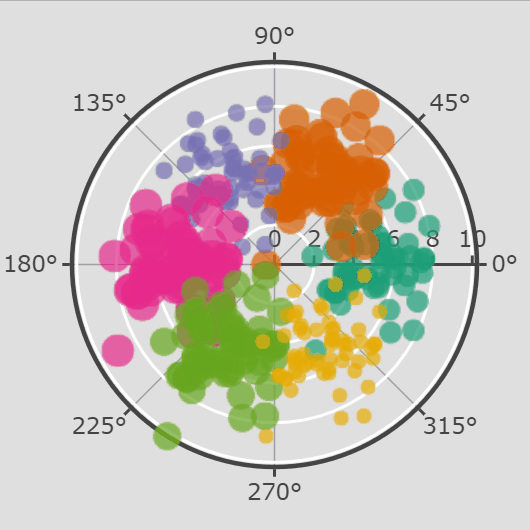
Creating a circular color gradient in Python is a great way to add a unique touch to your data visualization or graphic design project. With the right knowledge and tools, you can make a circular color gradient with ease in Python. This post will show you how to do it quickly and easily.
Steps to Make a Circular Color Gradient in Python
Step 1: Install the Python Imaging Library (PIL)
The first step in creating a circular color gradient in Python is to install the Python Imaging Library (PIL). PIL is an open source library that helps you work with images. To install PIL, open a terminal window and run the following command:
pip install Pillow
Step 2: Create a Color Map
The next step is to create a color map. A color map is a way of mapping colors to a particular range of values. To create a color map, use the following code:
from PIL import Image import numpy as np # Create a color map cm = np.array([[0, 0, 0], [255, 255, 0], [255, 0, 0], [0, 255, 0], [0, 0, 255]]) # Create an image im = Image.fromarray(cm)
Step 3: Rotate the Color Map
Now we need to rotate the color map so that it forms a circle. To do this, we can use the rotate() function in PIL. The following code will create a rotated color map:
# Rotate the color map im = im.rotate(45)
Step 4: Create a Circular Color Gradient
Now we can create a circular color gradient using the color map we just created. To do this, use the following code:
# Create a circular color gradient im = im.point(lambda x: x * 360) # Save the result im.save('circular_gradient.png')
Step 5: View the Result
The final step is to view the result of our circular color gradient. To do this, open the image in an image viewer and you should see something like this:
People Also Ask
How do I create a radial color gradient in Python?
Creating a radial color gradient in Python is similar to creating a circular color gradient. The main difference is that the color map needs to be rotated by the angle of the radius instead of the angle of the circle. The rest of the steps are the same.
Can I create a color gradient in Python without using PIL?
Yes, you can create a color gradient in Python without using PIL. The Matplotlib library is a popular alternative to PIL and can be used to create a color gradient in Python.
What is the best way to create a color gradient in Python?
The best way to create a color gradient in Python depends on your specific needs. If you are looking for a library that is easy to use and has a wide range of features, then PIL is a good choice. If you are looking for a library with more advanced features, then Matplotlib is a better choice. Ultimately, the best way to create a color gradient in Python is the one that meets your needs.
What can I do with a color gradient in Python?
There are a variety of things you can do with a color gradient in Python. You can use it to create data visualizations, add unique touches to graphics, and much more. The possibilities are endless.
Can I create a 3D color gradient in Python?
Yes, you can create a 3D color gradient in Python. To do this, you will need to use a library such as Matplotlib, which has advanced 3D plotting capabilities.
Creating a circular color gradient in Python is a great way to add a unique touch to your data visualization or graphic design project. With the right knowledge and tools, you can make a circular color gradient with ease in Python. Following the steps outlined in this post, you can quickly and easily create a unique circular color gradient in Python.